Top 4 Ways to Create JavaScript Multiline Strings
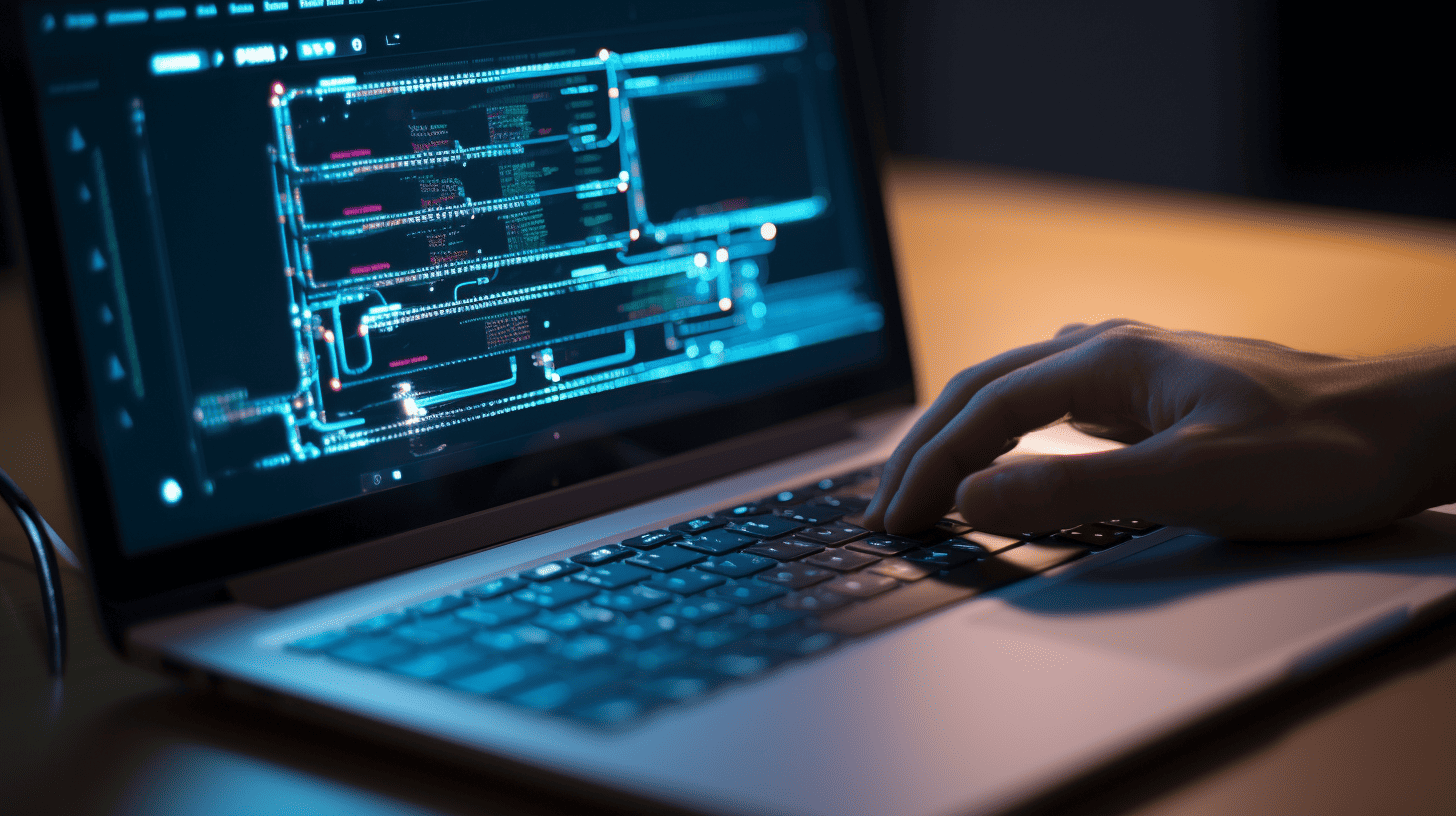
Did you ever need to work with long strings in JavaScript? Did you feel the code looks like a mess, whatever you try? Well, you’re not alone. It’s time you learned the ropes.
In this handy guide, you’ll discover four straightforward ways to create multiline strings in JavaScript. We’ll untangle the complexities, simplifying the process for you.
So, let’s dive right in and make your coding journey a lot smoother.
Key Takeaways
- JavaScript multiline strings make code easier to read and maintain.
- Multiline strings are handy when dealing with large blocks of text and eliminate the need for writing multiple strings separated by “+”.
- Template literals in ES6 provide a more efficient and cleaner way to create multiline strings.
- Backtick operators (
` `
) allow for easier formatting and multiline strings without escape sequences.
What are JavaScript Multiline Strings?
JavaScript Multiline Strings is a way to write a string that spans multiple lines in your code, making it easier to read and maintain.
Understanding the use and implementation of multiline strings can significantly enhance your coding efficiency and neatness.
Why Do You Need to Know JavaScript Multiline Strings?
Multiline strings are handy when you’re dealing with large blocks of text. Instead of writing multiple strings separated by “+”, you can efficiently handle text over several lines. This not only makes your code cleaner but also improves readability.
There are several ways to create a multi-line. The traditional method uses a backslash at the end of a line. However, the introduction of template literals in ES6 now allows you to create JavaScript multiline strings using backticks. This new method is more efficient and cleaner, making it a popular choice among developers.
How to Create JavaScript Multiline Strings?
You can create multiline strings in JavaScript in several ways.
One approach is by using template literals, which allow you to embed expressions and create multiline strings easily.
Other methods include newline escaping, string concatenation, and array joining – each offering unique benefits.
1. Use Template Literals
Template Literals are an efficient, clean, well-documented way to create a multiline string. When you use template literals, you’re making your code more readable and less prone to errors.
Here’s a quick example: Instead of concatenating strings and inserting new line characters, you can wrap your text in backticks (`). This allows your JavaScript multiline string to span multiple lines without extra fuss.
const multiLineString = `Apples
Bananas
Cherries`;
console.log(multiLineString);
/*
Outputs:
Apples
Bananas
Cherries
*/
But what can you do if you want your code to have a multiline string but the output to include no newline breaks? In that case, use the Newline Escaping character to escape the newlines.
const multiLineString = `Apples \
Bananas \
Cherries`;
console.log(multiLineString);
/*
Outputs:
Apples Bananas Cherries
*/
Template Literals have the added benefit of allowing string interpolation in JavaScript. You can read more on that further down the article.
Template Literals are supported in all modern browsers. Unless you need to support Internet Explorer (my heart is with you), you should look no further.
So, next time you’re coding, remember to use template literals. Not only will they make your code cleaner, but they’ll also reduce the time you spend debugging. Plus, they’re a modern feature of JavaScript worth incorporating into your toolkit.
2. Use Newline Escaping
Often, you’ll use newline escaping to create multiline strings in JavaScript, as it’s a commonly employed technique. Understanding this method is crucial for creating javascript multiline strings.
Here’s how it works: you end a line with a backslash (\
), and JavaScript interprets the following line as a continuation of the current string.
const multiLineString = "Apples \
Bananas \
Cherries";
console.log(multiLineString);
/*
Outputs:
Apples Bananas Cherries
*/
This use of newline escaping can be convenient when dealing with large chunks of text or when you’re looking to maintain the readability of your code. However, note that each newline and indentation will be part of your final string, which might not always be desired.
If you want to include new lines in your output text with this approach, you must explicitly add the new line character to your code.
const multiLineString = "Apples\n\
Bananas\n\
Cherries";
console.log(multiLineString);
/*
Outputs:
Apples
Bananas
Cherries
*/
Overall, mastering newline escaping is a vital skill for effectively creating multiline strings in JavaScript.
3. Use String Concatenation
How can string concatenation help in creating multiline strings in JavaScript?
It’s simple. JavaScript lets you concatenate or combine strings using the “+” operator. You can use this to create a multiline string. Start with a string, optionally add a newline character, and add another string. Repeat this as many times as you need.
Here’s an example:
const multiLineString = "Apples " +
"Bananas " +
"Cherries";
console.log(multiLineString);
/*
Outputs:
Apples Bananas Cherries
*/
If you prefer to output a multi-line string, you can do that too:
const multiLineString = "Apples\n" +
"Bananas\n" +
"Cherries";
console.log(multiLineString);
/*
Outputs:
Apples
Bananas
Cherries
*/
And that’s how you can use string concatenation to make multiline strings in JavaScript!
4. Use Array Joining
You’re now ready to explore array joining, another practical method for creating multiline strings in JavaScript. You’ll find it’s a cleaner, more efficient way to create multiline strings than string concatenation.
First, you’ll need an array. Populate it with the lines of your multiline string. When you’ve got your array set up, you’ll use array joining to merge the elements into a single string. Call the join method on your array, and provide the newline character as the argument. This tells JavaScript to add a new line between each array element when joining them together.
const lines = ["Apples",
"Bananas",
"Cherries"];
const multiLineString = lines.join("\n");
console.log(multiLineString);
/*
Outputs:
Apples
Bananas
Cherries
*/
Voila! With array joining, you’ve created a clean, well-documented multiline string. Try it and discover how array joining can streamline your JavaScript coding.
Key Considerations For Successfully Working with JavaScript Multiline Strings
When dealing with JavaScript multiline strings, it’s crucial to understand the role of backtick operators and escape sequences in ensuring correct code execution.
Backtick operators (` `) allow easier formatting, including creating multiline strings without escape sequences. However, escape sequences like \n
are still valuable for controlling special characters within your strings.
Remember that backticks and escape sequences are your friends for successfully working with JavaScript multiline strings – use them wisely.
Always test your code to ensure it’s running as expected. With practice, you’ll master these techniques and write efficient, clean, and well-documented code.
What are Strings in JavaScript?
In JavaScript, strings are sequences of characters used to represent text. They can be created using single quotes, double quotes, or backticks.
Each method has its use cases and quirks, which we’ll discuss next.
Single Quote Strings
Single quote strings are no different from double quote strings, except for the delimiter used. You can use single-quote strings and double-quote ones interchangeably. However, it’s better to maintain consistency within the same code base.
Single quote strings allow you to use double quotes within the string without escaping.
const str = 'My "quoted" string!'
console.log(str);
/*
Outputs:
My "quoted" string!
*/
You can use single quotes to create multiline strings. Start and end your string with a single quote, then use a backslash at the end of each line. It’s a handy trick, especially when dealing with longer text blocks.
const multiLineString = 'Apples \
Bananas \
Cherries';
console.log(multiLineString);
/*
Outputs:
Apples Bananas Cherries
*/
Remember, though, that JavaScript doesn’t automatically insert a space or newline character at the end of each line. You’ll need to add those yourself.
Double Quote Strings
You’ve already learned that Single quote strings are no different from double quote strings, except for the delimiter used.
The only difference is that double quote strings allow you to use single quotes within the string without escaping.
const str = "My 'quoted' string!"
console.log(str);
/*
Outputs:
My 'quoted' string!
*/
Backticks
Backticks allow multiline strings and enable variables and expressions embedded in a process known as String Interpolation.
Backticks, denoted by (` `), are vital when creating multi-line strings. This isn’t just about getting your code to look pretty. It’s about crafting efficient, clean, and well-documented JavaScript multiline strings.
Here’s the magic: backticks introduce template literals, a new form of output variable values. You can embed expressions within your string rather than breaking your strings and concatenating variables.
const item1 = "apples";
const item2 = "bananas";
const item3 = "cherries";
const interpolatedString = `I love ${item1}, ${item2} and ${item3}.`;
console.log(interpolatedString);
/*
Outputs:
I love apples, bananas and cherries.
*/
You can also combine string interpolation with multiline outputs.
const item1 = "apples";
const item2 = "bananas";
const item3 = "cherries";
const interpolatedString = `I love ${item1}, ${item2} and ${item3}.
They are delicious!`;
console.log(interpolatedString);
/*
Outputs:
I love apples, bananas and cherries.
They are delicious!
*/
You’ll find that using backticks is a cleaner, more efficient method.
How to Create Multiline Strings in TypeScript?
Creating multiline strings in TypeScript is no different than creating them in JavaScript. TypeScript is a superset of JavaScript; as such, all valid JavaScript code is also valid TypeScript code as well.
const item1: string = "apples";
const item2: string = "bananas";
const item3: string = "cherries";
const interpolatedString: string = `I love ${item1}, ${item2} and ${item3}.
They are delicious!`;
console.log(interpolatedString);
/*
Outputs:
I love apples, bananas and cherries.
They are delicious!
*/
There is no TypeScript-specific way to create multiline strings.
Frequently Asked Questions
How Can I Convert Javascript Multiline Strings to Single Line Strings?
To convert a multiline string to a single line string in JavaScript, you can use the replace()
method with a regular expression to replace all newline characters (\n
) and carriage return characters (\r
) with an empty string or a space. Here’s how you can do it:
let multiLineString = `Apples
Bananas
Cherries`;
let singleLineString = multiLineString.replace(/\r?\n|\r/g, ' ');
console.log(singleLineString);
/*
Outputs:
Apples Bananas Cherries
*/
Can Javascript Multiline Strings Be Used With Arrays?
Yes, you can use multiline strings with arrays in JavaScript. You’d define your array and include the multiline strings as elements. Remember, they’re just strings, broken over several lines for readability.
let fruits = ['Apples', 'Bananas', 'Cherries'];
let multiLineString = fruits.join('\n');
console.log(multiLineString);
/*
Outputs:
Apples
Bananas
Cherries
*/
What’s the Difference Between Multiline Strings in Javascript and Python?
In JavaScript, you use backticks to create multiline strings, while in Python, you utilize triple quotes. It’s like comparing apples to oranges; each has its unique way of breaking lines in a string.
Can You Use Javascript Multiline Strings in JSON Objects?
No, you can’t use JavaScript multiline strings in JSON objects. JSON strictly follows a specific syntax, and multiline strings aren’t part of it. You’ll need to handle strings differently when working with JSON.
Conclusion
In conclusion, mastering JavaScript multiline strings is like learning to ride a penny-farthing in a world of mountain bikes. It’s an old-school skill, but it still holds its charm.
Remember, the key to nailing it is understanding its constraints and leveraging its potential. With patience and practice, you’ll be able to weave intricate lines of code quickly.
This is your stepping stone to becoming a proficient coder. Keep coding, and keep exploring!