What Is the JavaScript Double Question Marks (??) Operator?
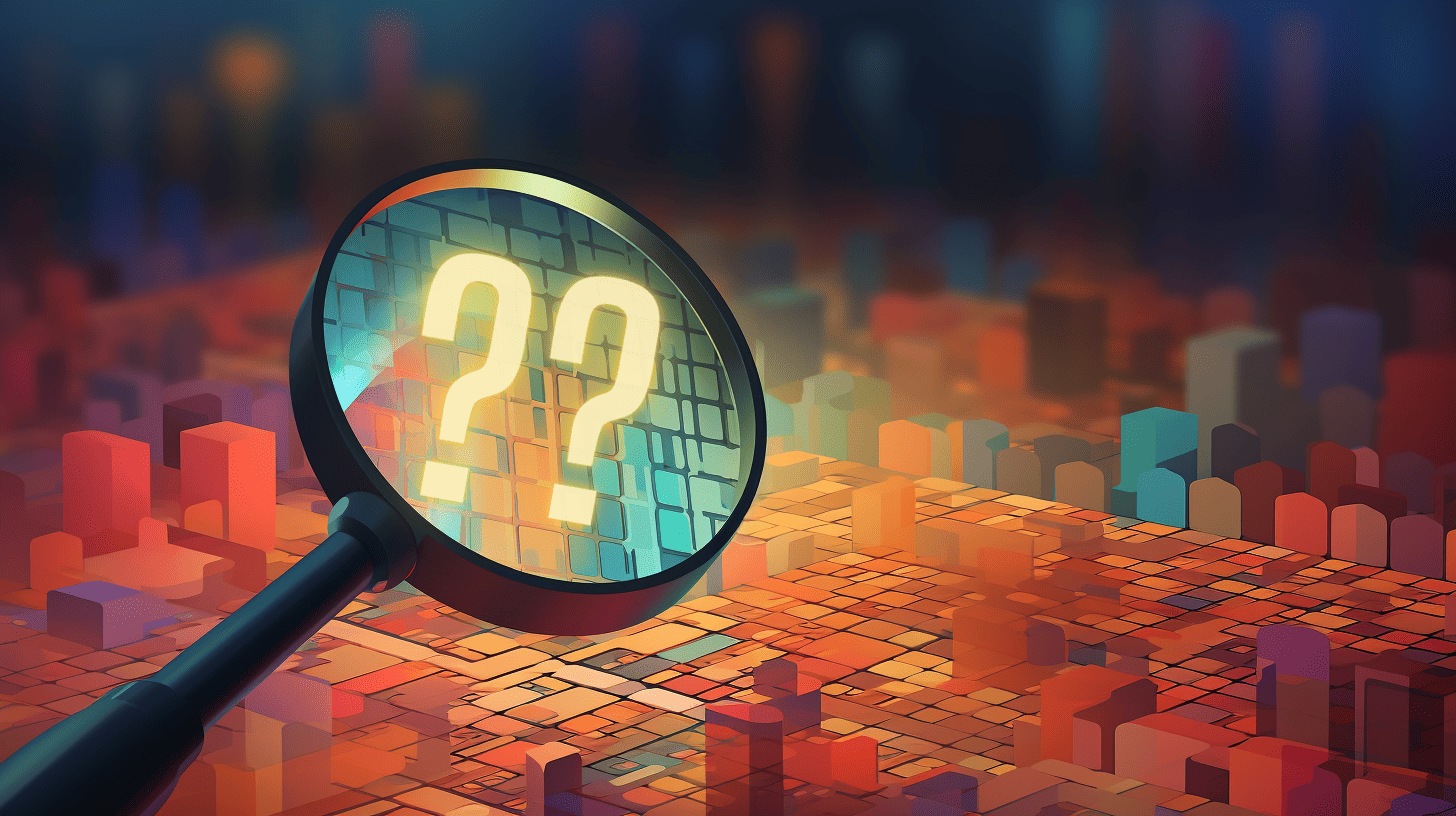
Have you ever encountered double question marks (??) in JavaScript code and wondered what they were? Did someone tell you about the Nullish Coalescing Operator, and you didn’t know what it meant? Or were you just wondering when you can use this operator to make your code more readable? Well, wonder no more.
You’re about to demystify JavaScript’s double question marks (??), also known as the Nullish Coalescing Operator. It may seem odd, but it’s extremely handy when working with potential null or undefined values.
It allows you to use the default value and prevent unexpected code behaviors.
Brace yourself, as you’ll learn how it interacts with the optional chaining operator and when to use it effectively.
By the end, you’ll be a pro at using this powerful operator in JavaScript.
Key Takeaways
- The nullish coalescing operator returns a default value when the left-hand side operand is null or undefined.
- It helps provide fallback values in case of null or undefined values.
- The nullish coalescing operator has higher precedence than the conditional (ternary) operator.
- It can be combined with the optional chaining operator to access a property with a fallback value safely.
- It can be replaced with the nullish coalescing assignment operator (??=) to make the initialization code even more concise.
What Is JavaScript Double Question Marks (??) Operator?
This JavaScript Double Question Marks operator kicks in to provide a default value when the left-hand side operand, or the first operand, turns out to be null
or undefined
. This is especially useful when dealing with variables that may not have been initialized.
Below is an example that translates the above behavior into JavaScript code.
const firstName = null;
const lastName = undefined;
const suffix = "Junior"
const nickname = "SuperCoder";
// If firstName is null or undefined, use the value of nickname
let displayName = firstName ?? nickname;
console.log(displayName); // Output: SuperCoder
// If lastName is null or undefined, use the value of nickname
displayName = lastName ?? nickname;
console.log(displayName); // Output: SuperCoder
// If suffix is null or undefined, use the hard-coded value
displayName = suffix ?? "";
console.log(displayName); // Output: Junior
If you were to write the above code using standard if
statements, it would become much more lengthy and unreadable. The example below uses an if
statement and the ternary operator to accomplish the same result.
const firstName = null;
const lastName = undefined;
const nickname = "SuperCoder";
// Here's how you would do the above checks without the nullish coalescing operator
let displayName;
if (firstName !== null && firstName !== undefined) {
displayName = firstName;
} else {
displayName = nickname;
}
console.log(displayName); // Output: SuperCoder
displayName = (lastName !== null && lastName !== undefined) ? lastName : nickname;
console.log(displayName); // Output: SuperCoder
The double question marks operator offers a reliable, concise way to set default values and avoid errors in your code. Remember, it’s all about preventing those pesky null
or undefined
values from causing havoc in your JavaScript programs.
What is the JavaScript Null Coalescing Assignment (??=) Operator?
The Nullish Coalescing Assignment (??=
) operator is a logical operator that only assigns a new value to a variable if that variable is null
or undefined
. It’s a shorthand for the Null Coalescing Operator (??
) that can be pretty useful in many situations where a default value must only be set if a variable hasn’t already been initialized.
Here’s a basic example demonstrating the ??=
operator:
let firstName = getFirstName();
firstName ??= "SuperCoder";
This operator is convenient when handling missing function arguments. I’ll cover this scenario in the section below.
When Should I Use the JavaScript Double Question Marks Operator?
You should use the JavaScript double question marks operator when you want to assign a default value in case the initial value is null
or undefined
.
It’s convenient when you’re accessing properties of an object that might not exist.
This operator allows you to write cleaner, more efficient code, reducing the risk of unexpected behaviors caused by null
or undefined
values.
Default Value Assignment
In the context of default value assignment, it’s beneficial to use the JavaScript double question marks operator when you want to assign a fallback value to a variable that might be null
or undefined
. With the double question marks operator, you can streamline this process.
const result = yourVariable ?? 'defaultValue';
If yourVariable
is null
or undefined
, result
will automatically be set to defaultValue
. It’s a more efficient, readable way to handle default value assignments in JavaScript.
Handling Missing Function Arguments
You can use the JavaScript double question marks operator to handle missing function arguments within the function body.
function myFunction(argA, argB) {
argA = argA ?? 'A';
argB = argB ?? 'B';
// Do something
}
The above code can be shortened even further using the Null Coalescing Assignment:
function myFunction(argA, argB) {
argA ??= 'A';
argB ??= 'B';
// Do something
}
This allows you to write code that provides more predictable results and minimizes edge cases where function callers can’t or won’t provide all the arguments.
Accessing Object Properties
Interestingly, when accessing object properties that might be undefined, it’s much safer to use the JavaScript double question marks operator combined with the Optional Chaining Operator (?.
).
Suppose you have a complex object, and you’re unsure whether a property exists or not. Instead of risking a TypeError, you utilize the optional chaining operator to access properties. However, you’d get an undefined result if the property is undefined.
That’s where the double question marks come into play. You can set a default value that’ll be returned if the property is undefined.
const user = {
profile: {
name: "John Doe",
age: 30,
address: {
city: "New York",
country: "USA"
}
}
};
// Safe access to deeply nested properties using optional chaining and nullish coalescing
const city = user?.profile?.address?.city ?? "Default City";
console.log(city); // Output: New York
// If any of the nested properties are null or undefined, the default value will be used
const postalCode = user?.profile?.address?.postalCode ?? "00000";
console.log(postalCode); // Output: 00000
Using the double questions mark operator is a clean, concise way to ensure you access object properties safely in JavaScript.
Why JavaScript Needed the Double Question Marks Operator?
JavaScript needed the double question marks operator to provide a robust solution for handling the two specific cases of null
and undefined
values.
Before the introduction of the Nullish Coalescing Operator (??
), developers often used the logical OR (||
) operator to provide default values. However, this approach has a significant drawback: it considers all falsy values (false
, 0
, ''
, NaN
, null
, undefined
) as equivalent for the purposes of providing a default value. In many cases, this behavior isn’t desired, as false
, 0
, empty string, etc., are legitimate values that shouldn’t trigger the use of a default value.
The following code illustrates the drawbacks of using a logical OR:
const options = {
isPrivate: false,
maxParticipants: 0,
};
// Using the logical OR (`||`) operator:
const isPrivate = options.isPrivate || true; // undesired result: true
const maxParticipants = options.maxParticipants || 10; // undesired result: 10
console.log(isPrivate); // Output: true
console.log(maxParticipants); // Output: 10
// Using the nullish coalescing operator (`??`):
const isPrivateCorrect = options.isPrivate ?? true; // desired result: false
const maxParticipantsCorrect = options.maxParticipants ?? 10; // desired result: 0
console.log(isPrivateCorrect); // Output: false
console.log(maxParticipantsCorrect); // Output: 0
In this example, isPrivate
and maxParticipants
are valid options with falsy values (false
and 0
, respectively). When using the ||
operator to provide default values, these falsy values are wrongly interpreted as triggers for default values.
With the ??
operator, the original falsy values are correctly preserved, as ??
only considers null
or undefined
as triggers for default values. This allows for more accurate, intuitive, and safer default value assignment, making the ??
operator a valuable addition to the language for developers.
Frequently Asked Questions
How Does the Nullish Coalescing Operator Differ From the Logical OR Operator in Javascript?
JavaScript’s Nullish Coalescing Operator (??
) differs from the logical OR (||
) by only returning the right operand if the left one is null
or undefined
, not on other falsy values like 0 or ""
.
What Is the Operator Precedence of the Nullish Coalescing Operator in Javascript?
In JavaScript, the Nullish Coalescing Operator (??
) ranks third-lowest in operator precedence. It’s lower than logical AND (&&
), has the same precedence as the logical OR (||
), but higher priority than the assignment (=
) and ternary (?:
) operators.
To indicate precedence with JavaScript’s Nullish Coalescing Operator, use parentheses. Here are a few examples.
let a = false;
let b = null;
let c = true;
// Using parentheses to indicate precedence:
let result = (a ?? b) || c; // evaluates as (false) || true = true
console.log(result); // Output: true
result = a ?? (b || c); // evaluates as false ?? (null || true) = false ?? true = false
console.log(result); // Output: false
How Does the Nullish Coalescing Operator Handle Non-Null and Non-Undefined Values?
If you’re dealing with non-null and non-undefined values, the JavaScript double question marks (??
) operator returns the left-hand value. It’s only when it’s null
or undefined
, it defaults to the right-hand side value.
Can the Nullish Coalescing Operator Be Combined With Other Operators Besides the Optional Chaining Operator?
You can combine the nullish coalescing operator (??) with other operators. However, due to its relatively low precedence, you’ll need to use parentheses to dictate the order of operations to avoid unexpected results.
You should note that this operator doesn’t have associativity with other operators, which could cause exceptions. For example:
let a = false;
let b = null;
let c = true;
// Due to the lack of associativity, this will throw a SyntaxError
result = a ?? b || c;
Is the Double Question Marks Operator Available in TypeScript?
The double question marks operator has been available in TypeScript since version 3.7. Here is an example of code usage.
type User = {
name: string | null;
age?: number;
};
const user: User = {
name: null,
};
// Use the nullish coalescing operator to provide default values
const userName: string = user.name ?? 'Default Name';
const userAge: number = user.age ?? 18;
console.log(userName); // Output: Default Name
console.log(userAge); // Output: 18
Conclusion
In conclusion, the JavaScript double question marks operator is a versatile tool in your coding arsenal. It helps handle null
or undefined
values seamlessly, assigning default values and preventing unexpected behaviors.
Coupled with the optional chaining operator, it ensures safe access to object properties.
So, dive in, practice, and use this operator in your JavaScript or TypeScript coding experience. You can also learn other handy tricks, such as working with multiline strings in JavaScript.
It’s time to code smarter, not harder!
What an excellent extensive post here! Great examples. Although I was already aware of the drawbacks of using the OR || operator, your article really goes in depth with pleasant snippets and flowcharts to look at! What I really learned from your post (what I was initially googling for) was the benefits of using the ??= null coalescing assignment operator in specific use cases.
I can see you dedicated quite a significant effort and passion writing those articles!
Thanks!